챌린지 1일차 학습 정리
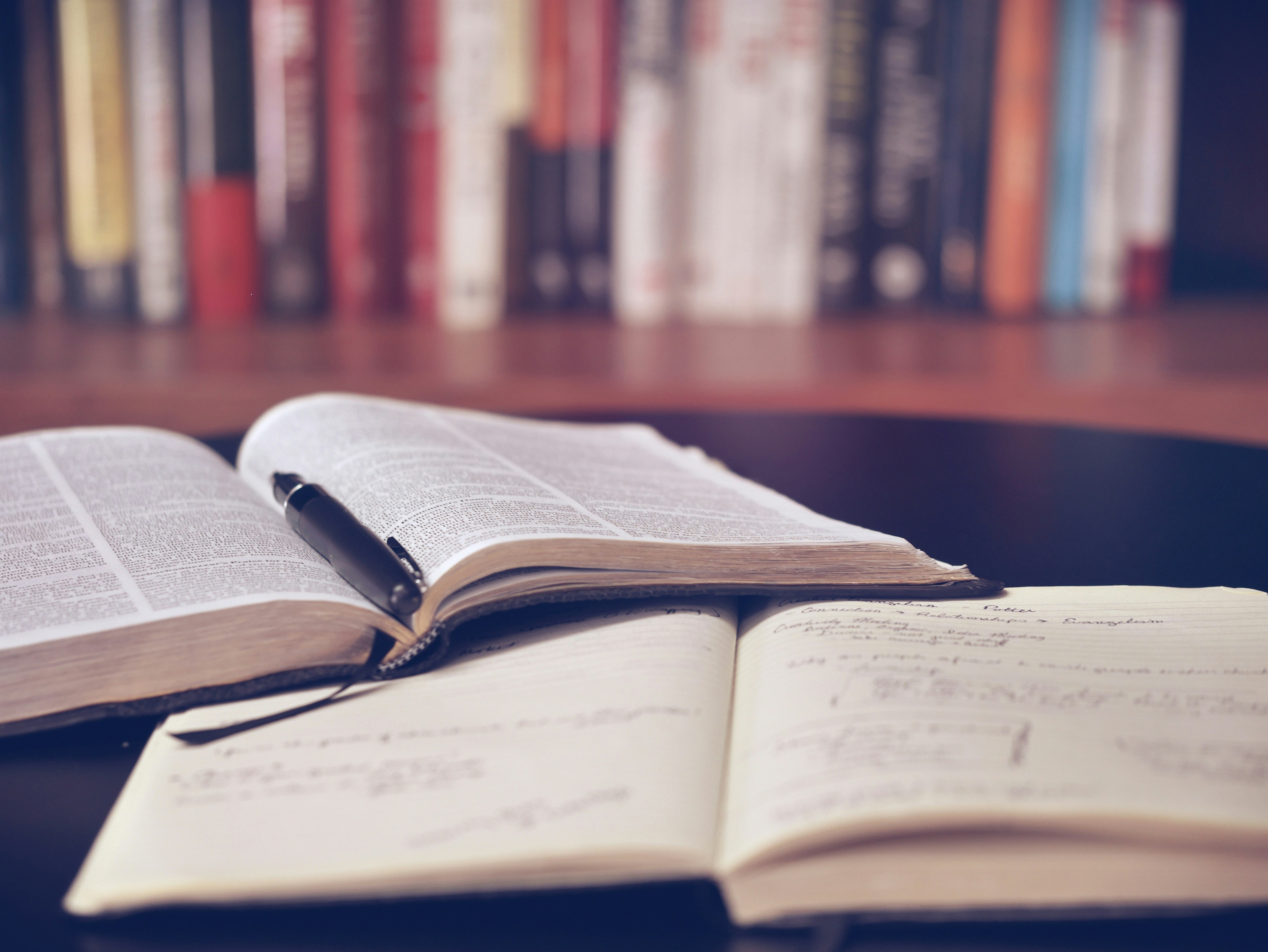
네이버 부스트캠프 9기 챌린지 1일차 학습 정리 페이지입니다.
- 누군가 작성한 것을 그대로 쓰는 것이 아니라 나만의 언어로 재구조화하여 작성해야 합니다.
- 기술 키워드에 대한 상세 내용도 좋고, 미션 해결 과정에서 기능 구현을 성공한 사례도, 트러블 슈팅 경험도 좋습니다.
목차
학습한 내용
Git 기초 명령어
Feature | Command | Description |
---|---|---|
[Create a Repository] | ||
git clone [repository_url] | Download from an existing repository | |
[Observe your Repository] | ||
git status | List new or modified files not yet committed | |
git diff | Show the changes between two commits ids | |
git blame [file] | List the change dates and authors for a file | |
[Working with Branches] | ||
git branch new_branch | Create a new branch called new_branch | |
[Make a change] | ||
git commit -am “commit message” | commit all your tracked files to versioned history | |
git reset –hard | Revert everything to the last commit | |
git revert -m | Revert merge commit | |
[Synchronize] | ||
git fetch | Get the latest changes from origin | |
git pull –rebase | Get the latest changes from origin |
JSDoc 기초 사용법
JSDoc 주석은 /** ... */
사이에 기술합니다.
/**
* [설명 부분]
*
* @param {number} param1 [파라미터 설명]
* @param {string} param2 [파라미터 설명]
* @returns {boolean} [반환 값 설명 부분]
*/
const solution = (param1, param2) => {
...
return true;
}
설명 부분
: 함수나 클래스 등에 대한 설명을 지정하는 부분입니다.@param
: 파라미터의 타입과 설명을 지정하는 부분입니다.@returns
: 반환 값의 타입과 설명을 지정하는 부분입니다.
자바스크립트 표준 입력 방법
const readline = require("readline");
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout,
});
rl.on("line", (line) => {
...
rl.close();
});
rl.on("close", () => {
console.log("프로그램 종료");
});
"line"
: 표준 입력을 한 줄로 입력받는 부분입니다."close"
: 표준 입력이 종료될 경우 실행하는 코드를 담는 부분입니다.rl.close()
: 입력 중단을 선언하는 부분입니다. 해당 코드가 존재하지 않으면 사용자가Ctrl + C
를 누르기 전까진 반복해서 표준 입력을 받습니다.